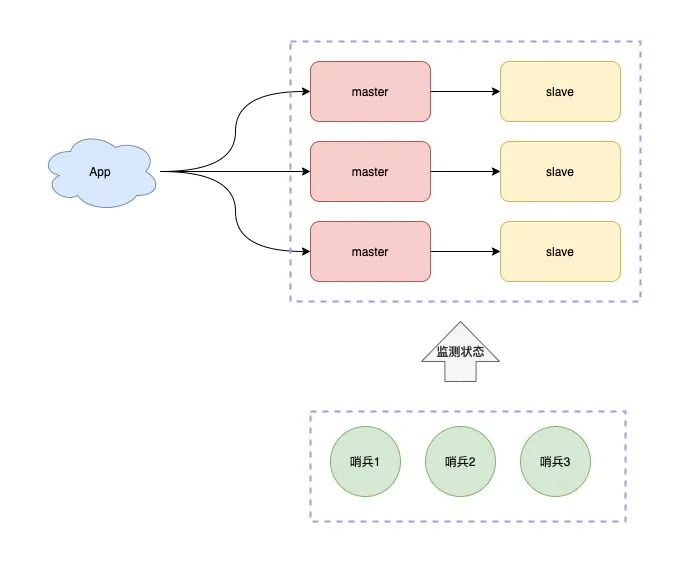
导入依赖
<!--Springboot集成 Redis ,Springboot2.x默认是用Lettuce连接redis,Lettuce可伸缩,线程安全-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
配置applcation.yml
# redis 配置
redis:
server:
pattern: cluster
nodes: 127.0.0.1:3000,127.0.0.1:3001,127.0.0.1:3002,127.0.0.1:3003,127.0.0.1:3004,127.0.0.1:3005
password:
maxIdle: 8
minIdle: 4
maxTotal: 8
maxWaitMillis: 6000
timeOut: 6000
方式一:redis(存储各种对象)
@Configuration
public class RedisConfig {
@Value("${redis.server.nodes}")
private String redisServerNodes;
@Value("${redis.server.password}")
private String redisServerPassword;
@Bean
public RedisClusterConfiguration getRedisClusterConfiguration() {
RedisClusterConfiguration redisClusterConfiguration = new RedisClusterConfiguration();
String[] serverArray = redisServerNodes.split(",");
Set<RedisNode> nodes = new HashSet<RedisNode>();
for (String ipPort : serverArray) {
String[] ipAndPort = ipPort.split(":");
nodes.add(new RedisNode(ipAndPort[0].trim(), Integer.parseInt(ipAndPort[1])));
}
redisClusterConfiguration.setClusterNodes(nodes);
RedisPassword pwd = RedisPassword.of(redisServerPassword);
redisClusterConfiguration.setPassword(pwd);
return redisClusterConfiguration;
}
//指定redisTemplate类型,如下为<String, Object>
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate();
template.setConnectionFactory(redisConnectionFactory);
// 使用JSON格式序列化对象,对缓存数据key和value进行转换
Jackson2JsonRedisSerializer<Object> jacksonSeial = new Jackson2JsonRedisSerializer<>(Object.class);
// 解决查询缓存转换异常的问题
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
objectMapper.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jacksonSeial.setObjectMapper(objectMapper);
// 设置RedisTemplate模板API的序列化方式为JSON
template.setDefaultSerializer(jacksonSeial);
return template;
}
}
//1.指定存储类型
@Autowired
private RedisTemplate<String,String> redisTemplate;
//2.未指定存储类型
@Autowired
private RedisTemplate redisTemplate;
//存储
redisTemplate.opsForValue().set(keyName, valueObject, Duration.ofXXX(n));
//Duration.ofNanos() 纳秒
//Duration.ofMillis() 毫秒
//Duration.ofSeconds() 秒
//Duration.ofMinutes() 分钟
//Duration.ofHours() 小时
//Duration.ofDays() 天
//查询
redisTemplate.opsForValue().get(keyName);
方式二:redission (常用于储存分布式锁)
@Configuration
public class RedissonConfig {
@Value("${redis.server.pattern:single}")
private String pattern;
@Value("${redis.server.host:127.0.0.1}")
private String host;
@Value("${redis.server.nodes:1}")
private List<String> nodes;
@Value("${redis.server.port:6379}")
private String port;
@Value("${redis.server.password}")
private String password;
@Value("${redis.server.database:1}")
private Integer database;
@Bean
@ConditionalOnProperty(prefix = "redis.server", name = "pattern", havingValue = "cluster")
public RedissonClient clusterRedisClient() {
Config config = new Config();
ClusterServersConfig clusterServersConfig = config.useClusterServers();
if (!StringUtils.isBlank(password)) {
clusterServersConfig.setPassword(password);
}
// 集群状态扫描间隔时间,单位是毫秒
clusterServersConfig.setScanInterval(2000);
for (String node : nodes) {
String url = "redis://" + node;
clusterServersConfig.addNodeAddress(url);
}
RedissonClient redissonClient = Redisson.create(config);
return redissonClient;
}
@Bean
@ConditionalOnProperty(prefix = "redis.server", name = "pattern", havingValue = "single")
public RedissonClient singleRedisClient() {
Config config = new Config();
SingleServerConfig singleServerConfig = config.useSingleServer();
if (!StringUtils.isBlank(password)) {
singleServerConfig.setPassword(password);
}
String url = "redis://" + host + ":" + port;
singleServerConfig.setAddress(url);
singleServerConfig.setDatabase(database);
RedissonClient redissonClient = Redisson.create(config);
return redissonClient;
}
}
//锁工具封装
public class RedissionLockTools{
@Autowired
private static RedissonClient redissonClient;
//加锁
public static boolean lock(String lockName){
//声明key对象
String key = "LOCK_TITLE" + lockName;
//获取锁对象
RLock mylock = redissonClient.getLock(key);
//加锁,并且设置锁过期时间3秒,防止死锁的产生 uuid+threadId
mylock.lock(3, TimeUnit.SECONDS);
//加锁成功
return true;
}
//锁的释放
public static void unlock(String lockName) {
//必须是和加锁时的同一个key
String key = "LOCK_TITLE" + lockName;
//获取所对象
RLock mylock = redissonClient.getLock(key);
//释放锁(解锁)
mylock.unlock();
}
}
public Object getUserById(User user) throws IOException{
String lockName = "lockName01";
//加锁
RedissionLockTools.lock(lockName);
//执行具体业务逻辑
//~~~~~~~
//执行具体业务逻辑
//释放锁
RedissionLockTools.unlock(lockName);
//返回结果
return result;
}
声明:本站所有文章,如无特殊说明或标注,均为本站原创发布。任何个人或组织,在未征得本站同意时,禁止复制、盗用、采集、发布本站内容到任何网站、书籍等各类媒体平台。如若本站内容侵犯了原著者的合法权益,可联系我们进行处理。